In this tutorial, you will learn how to add page break in a PDF file using JavaScript and html2pdf.js. The complete source code of this project is given below.
To get started, add the CDN of html2pdf.js
library inside your HTML document.
<script src="https://cdnjs.cloudflare.com/ajax/libs/html2pdf.js/0.8.0/html2pdf.bundle.min.js"></script>
Basically, html2pdf.js
library has a special class html2pdf__pagebreak
that we can use in our HTML code to insert a page break inside PDF document. For example, we can create an empty <div>
element and add this class as mentioned below.
<div class="html2pdf__page-break"></div>
Complete html2pdf Page Break Example Code
Now, let’s have a look at the complete example of html2pdf page break.
index.html
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css"> <div id="element-to-print"> <span>I'm on page 1!</span> <div class="html2pdf__page-break"></div> <span>I'm on page 2!</span> </div> <script src="https://cdnjs.cloudflare.com/ajax/libs/html2pdf.js/0.8.0/html2pdf.bundle.min.js"></script> <script> var element = document.getElementById('element-to-print'); html2pdf(element, { margin: 10, filename: 'myfile.pdf', image: { type: 'jpeg', quality: 0.98 }, html2canvas: { scale: 2, logging: true, dpi: 192, letterRendering: true }, jsPDF: { unit: 'mm', format: 'a4', orientation: 'portrait' } }); </script>
It’s time to open the index.html
file inside the web browser. So, go ahead and open it.
When you open the index.html
file in browser, you will notice that a PDF file will be generated. Now, open that PDF file, you will see that it has two pages. The first page contains the content of first <span>
element whereas the second page will contain the content of second <span>
element.
html2pdf Page Break Example Screenshots
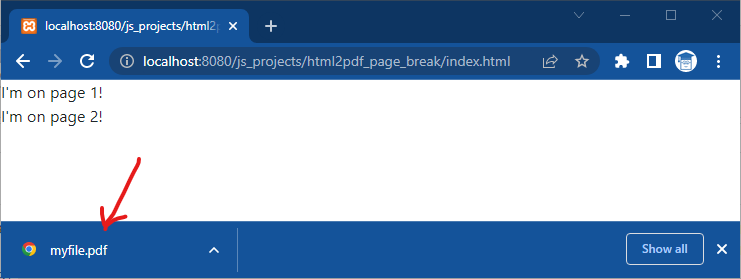
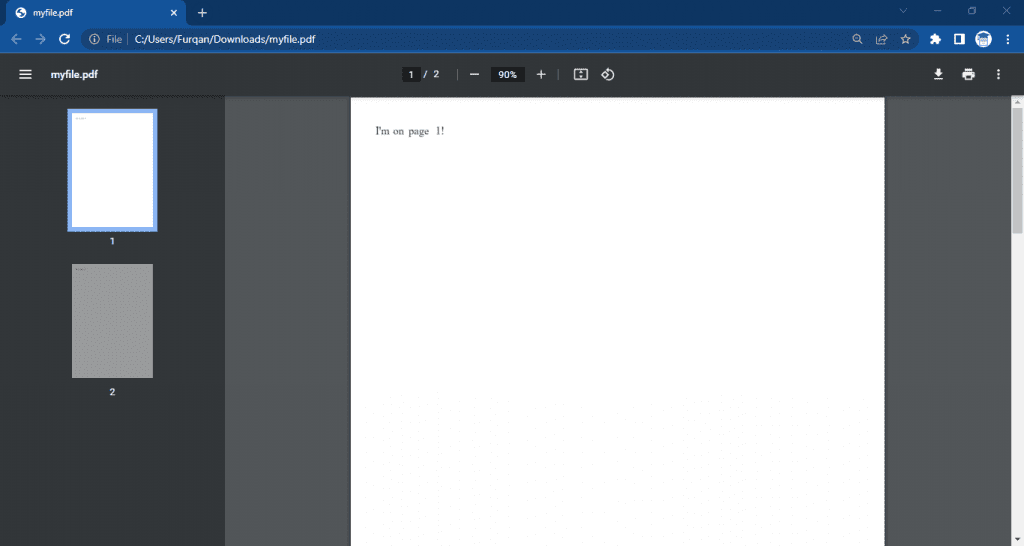
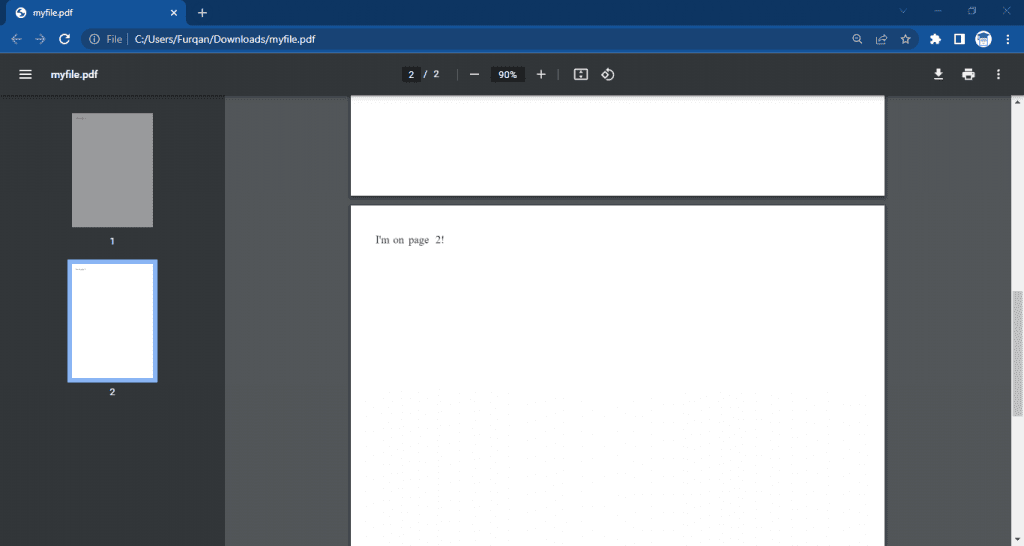